Subscribing to current font events ↩
This example shows a floating window that displays information about the current font. The window knows when the user has switched the current font and updates accordingly. The displayed information is updated when the data within the current font is changed.
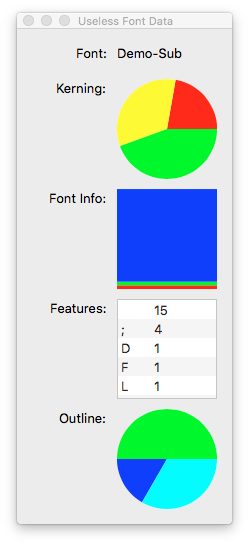
The tool uses the Subscriber module to subscribe to font kerning, info, features changed and to all outline changes. It also draws a small graph using merz
.
import time
import pathlib
from mojo.subscriber import Subscriber
import ezui
class FontEventLogSubscriber(Subscriber, ezui.WindowController):
debug = True
def build(self, font=None):
font = font.asDefcon()
self.font = font
content = """
filename.ufo @fileNameLabel
|--------------| @logTable
| when | event |
|--------------|
"""
descriptionData = dict(
fileNameLabel=dict(
text=pathlib.Path(font.path).name
),
logTable=dict(
width=400,
height=400,
columnDescriptions=[
dict(
identifier="when",
title="When",
width=60
),
dict(
identifier="event",
title="Event"
)
]
)
)
self.w = ezui.EZWindow(
content=content,
descriptionData=descriptionData,
controller=self
)
self.setAdjunctObjectsToObserve(
[
font,
font.info,
font.kerning,
font.groups,
font.features,
font.layers
]
)
def started(self):
self.w.open()
def destroy(self):
self.clearObservedAdjunctObjects()
def logEvent(self, name):
item = dict(
when=time.strftime("%H:%M:%S"),
event=name
)
table = self.w.getItem("logTable")
items = table.get()
items.append(item)
if len(items) > 100:
items.pop(0)
table.set(items)
table.scrollToIndex(len(items) - 1)
def fontDocumentWillClose(self, info):
if info["font"].asDefcon() == self.font:
self.w.close()
self.destroy()
def adjunctFontDidChange(self, info):
self.logEvent("adjunctFontDidChange")
def adjunctFontDidReloadGlyphs(self, info):
self.logEvent("adjunctFontDidReloadGlyphs")
def adjunctFontDidChangeGlyphOrder(self, info):
self.logEvent("adjunctFontDidChangeGlyphOrder")
def adjunctFontDidChangeGuidelines(self, info):
self.logEvent("adjunctFontDidChangeGuidelines")
def adjunctFontInfoDidChange(self, info):
self.logEvent("adjunctFontInfoDidChange")
def adjunctFontInfoDidChangeValue(self, info):
self.logEvent("adjunctFontInfoDidChangeValue")
def adjunctFontKerningDidChange(self, info):
self.logEvent("adjunctFontKerningDidChange")
def adjunctFontKerningDidChangePair(self, info):
self.logEvent("adjunctFontKerningDidChangePair")
def adjunctFontKerningDidClear(self, info):
self.logEvent("adjunctFontKerningDidClear")
def adjunctFontKerningDidUpdate(self, info):
self.logEvent("adjunctFontKerningDidUpdate")
def adjunctFontGroupsDidChange(self, info):
self.logEvent("adjunctFontGroupsDidChange")
def adjunctFontGroupsDidChangeGroup(self, info):
self.logEvent("adjunctFontGroupsDidChangeGroup")
def adjunctFontGroupsDidClear(self, info):
self.logEvent("adjunctFontGroupsDidClear")
def adjunctFontGroupsDidUpdate(self, info):
self.logEvent("adjunctFontGroupsDidUpdate")
def adjunctFontFeaturesDidChange(self, info):
self.logEvent("adjunctFontFeaturesDidChange")
def adjunctFontFeaturesDidChangeText(self, info):
self.logEvent("adjunctFontFeaturesDidChangeText")
def adjunctFontLayersDidChange(self, info):
self.logEvent("adjunctFontLayersDidChange")
def adjunctFontLayersDidChangeLayer(self, info):
self.logEvent("adjunctFontLayersDidChangeLayer")
def adjunctFontLayersDidSetDefaultLayer(self, info):
self.logEvent("adjunctFontLayersDidSetDefaultLayer")
def adjunctFontLayersDidChangeOrder(self, info):
self.logEvent("adjunctFontLayersDidChangeOrder")
def adjunctFontLayersDidAddLayer(self, info):
self.logEvent("adjunctFontLayersDidAddLayer")
def adjunctFontLayersDidRemoveLayer(self, info):
self.logEvent("adjunctFontLayersDidRemoveLayer")
def adjunctFontLayersDidChangeLayerName(self, info):
self.logEvent("adjunctFontLayersDidChangeLayerName")
font = CurrentFont()
FontEventLogSubscriber(font=font)