Draw reference glyph ↩
This example shows a very simple tool which displays the current glyph in a system font next to the glyph shape in the Glyph Editor using Subscriber
.
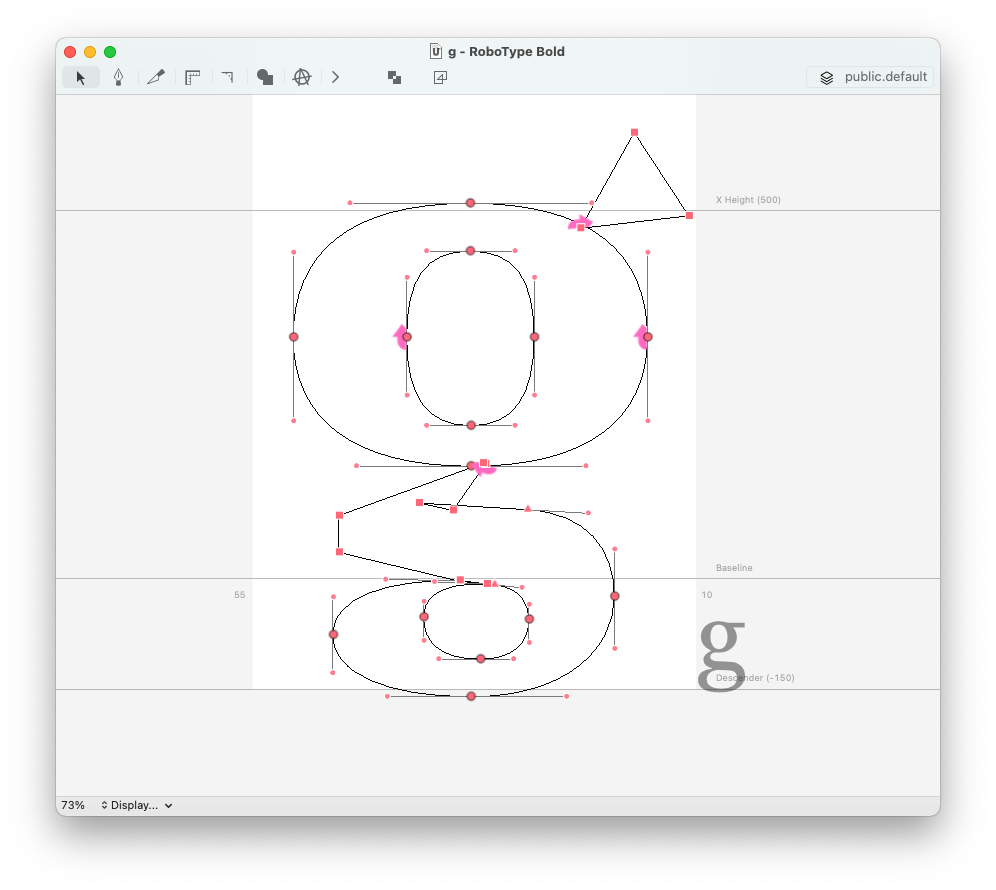
This is how the code works:
-
the tool subclasses Subscriber
- the tool overrides three Subscriber methods:
- with
build()
accesses the glyphEditor object and itsmerz
container - with
started()
creates a text line sublayer into themerz
container - with
destroy()
cleans the sublayers from the container once unregistered
- with
-
the tools uses the Subscriber
glyphEditorDidSetGlyph
callback to set the text attribute of the text line sublayer - note that the tool is initiated using a
registerGlyphEditorSubscriber
, so the tool will catch only events related to the glyph editor in use
from mojo.subscriber import Subscriber, registerGlyphEditorSubscriber
class DrawReferenceGlyph(Subscriber):
debug = True
def build(self):
glyphEditor = self.getGlyphEditor()
self.container = glyphEditor.extensionContainer(
identifier="com.roboFont.DrawReferenceGlyph.foreground",
location="foreground",
clear=True)
def started(self):
self.textLineLayer = self.container.appendTextLineSublayer(
pointSize=100,
font='Georgia',
text="",
fillColor=(0, 0, 0, 0.4),
horizontalAlignment="left"
)
def destroy(self):
self.container.clearSublayers()
def glyphEditorDidSetGlyph(self, info):
glyph = info["glyph"]
if glyph is None:
self.textLineLayer.setText("")
return
txt = ""
if glyph.unicode is not None:
# get character for glyph
txt = chr(glyph.unicode)
self.textLineLayer.setPosition((glyph.width, 0))
self.textLineLayer.setText(txt)
def glyphEditorGlyphDidChangeMetrics(self, info):
glyph = info["glyph"]
if glyph is None:
self.textLineLayer.setText("")
return
self.textLineLayer.setPosition((glyph.width, 0))
if __name__ == '__main__':
registerGlyphEditorSubscriber(DrawReferenceGlyph)