Proof character set ↩
This example shows how to create a simple character set proofer using DrawBot.
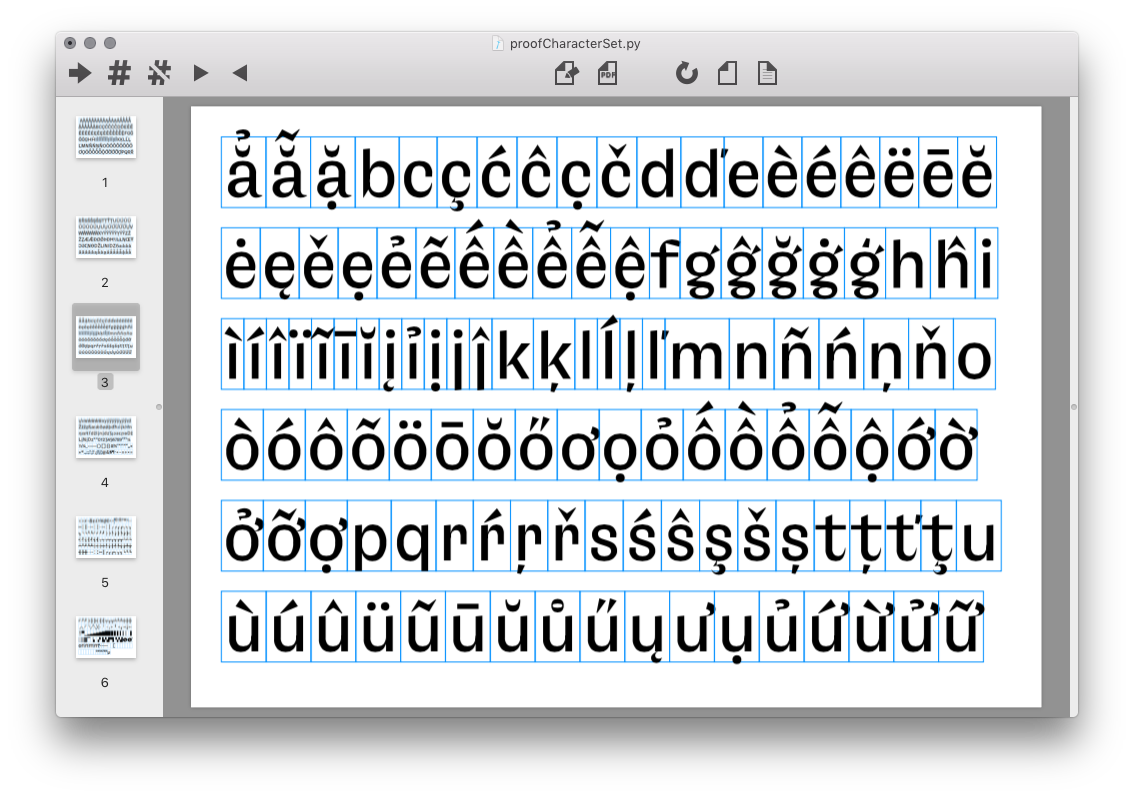
All glyphs in the font are displayed side-by-side following the order defined in font.glyphOrder
. Some basic parameters such as page margin and size/spacing of the glyph boxes can be adjusted using the variables at the top of the script.
'''Character Set Proofer'''
f = CurrentFont()
# main variables
boxHeight = 70
boxPaddingX = 0
boxPaddingY = 20
margin = 30
# calculate scale
s = float(boxHeight) / f.info.unitsPerEm
# define page size
size('A4Landscape')
pageWidth, pageHeight = width(), height()
# calculate initial positions
x = margin
y = pageHeight - margin - boxHeight
# draw glyphs
xBox, yBox = x, y
for i, glyphName in enumerate(f.glyphOrder):
g = f[glyphName]
boxWidth = g.width*s
# jump to next line
if xBox + boxWidth >= pageWidth - margin:
xBox = x
yBox -= boxHeight + boxPaddingY
# jump to next page
if yBox < margin:
yBox = y
newPage(pageWidth, pageHeight)
# draw glyph cell
stroke(0, 0.5, 1)
fill(None)
rect(xBox, yBox, boxWidth, boxHeight)
# draw glyph
xGlyph = xBox
yGlyph = yBox - f.info.descender*s
save()
translate(xGlyph, yGlyph)
fill(0)
stroke(None)
scale(s)
drawGlyph(g)
restore()
# move to next glyph
xBox += boxWidth + boxPaddingX
This example could be extended to show the font name and additional information for each glyph, such as the glyph name, unicode, left and right margins, width, guidelines, etc. Give it a try!